5 Things To Know About Swift Programming
- techwriting
- Nov 30, 2019
- 5 min read
Swift is a powerful and intuitive programming language for Apple macOS, iOS, watchOS, tvOS and beyond. Writing Swift code is interactive and fun, the syntax is concise yet expressive and Swift includes modern features that developers love. Swift code is safe by design and also produces software that runs lightning fast. Swift is the result of the latest research on programming languages, combined with decades of experience building Apple platforms. Here are some tips that you need to know if you want to be a successful iOS developer...
1. Introduction to Swift programming language
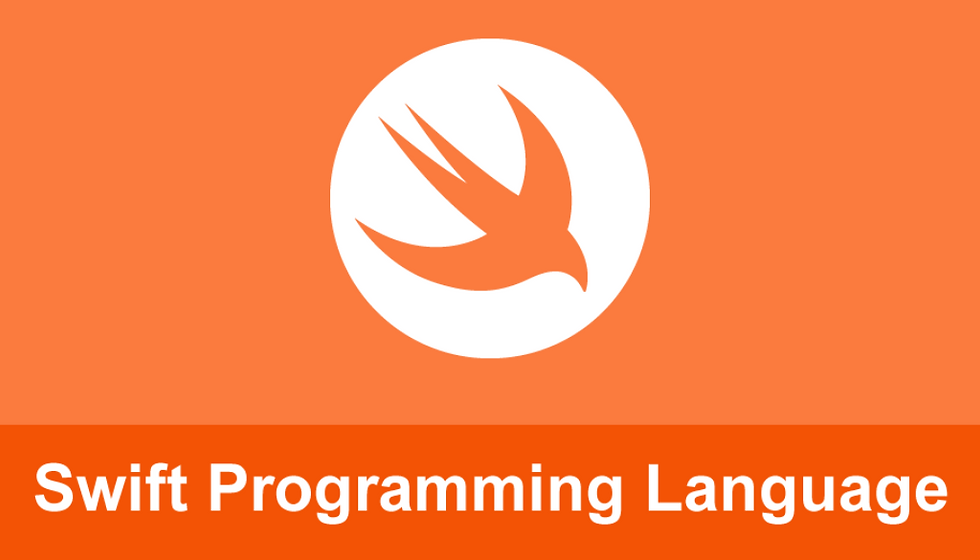
Swift is a new programming language designed by Apple for iOS and Mac OS development. It was designed to take the best of its predecessors, like C and Objective C.
Swift adopts safe programming patterns and adds modern features to make programming easier, more flexible and more fun. It is really easy to learn Swift programming and get used to it, which is great news if you're new to iOS development. If you are an experienced iOS developer, don't worry, the knowledge and expertise you have gained over the years won't go to waste your time.
In addition to the ability to write Swift code along side Objective-C code, it is important to mention that if you are an experienced developer that wants to continue using Objective-C as his/her primary language for Apple iOS development, it is definitely possible. The language is still fully supported and is expected to remain supported for the near and probably also far future.
2. Syntax
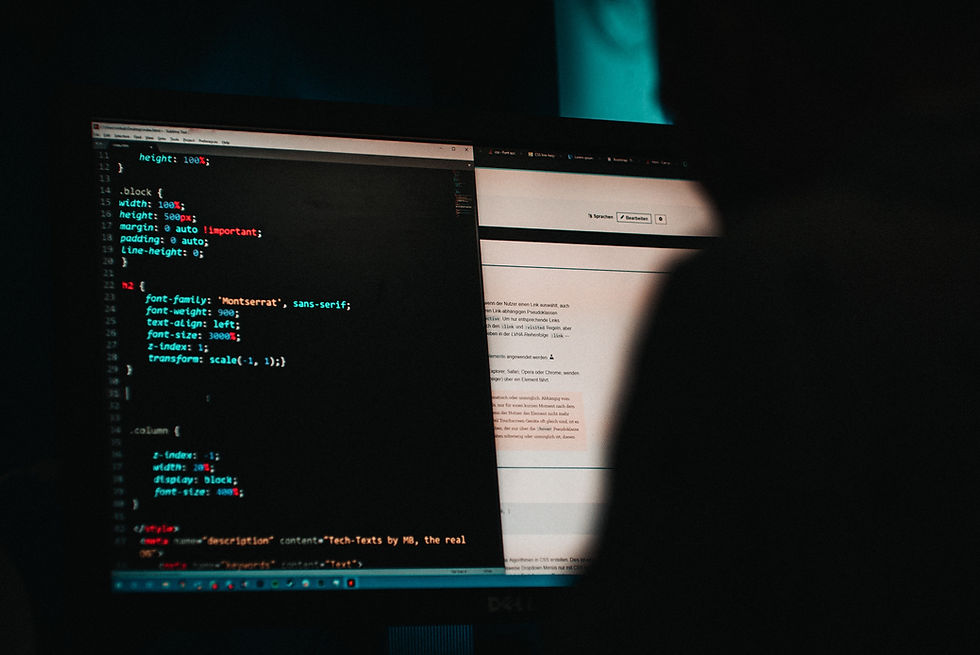
The first thing every programmer has to do when learning a new programming language is make a Hello World app. In Swift, this can be achieved using a single line:
- println("Hello World!")
If you type in this single line of code in the playground file you opened a moment ago, you will see the output for this statement on the right side of the screen. Notice that there is no semicolons at the end of the line. That is because in Swift, you don't need to write semicolons at the end of every statement.
Now that we have got that out of the way, we can dive in a little deeper into Swift's syntax. I recommend typing in the code samples in the playground file you recently created. Some of the code samples build upon previous ones, so it is important to go through all of them in order.
A few important things you should know about variables and constants in Swift:
1) A constant's value doesn't have to be known at compile time, but it can only be assigned a value once.
2) You don't have to specify the variable/constant's type. It can be inferred from the provided value.
3) Once a variable's type has been set (whether implicitly or explicitly), values assigned to it must conform to that type.
4) Similar to other languages you may be accustomed to, the array's first index is 0. And so on...
A few important things you should know about conditions and loops in Swift:
1) You don't have to use parentheses around the condition but you do have to include braces around the body.The conditional must a boolean.
2) This means that implicit comparisons to zero aren't supported, unlike what you may be used to from other languages. And so much more...
3. App structure
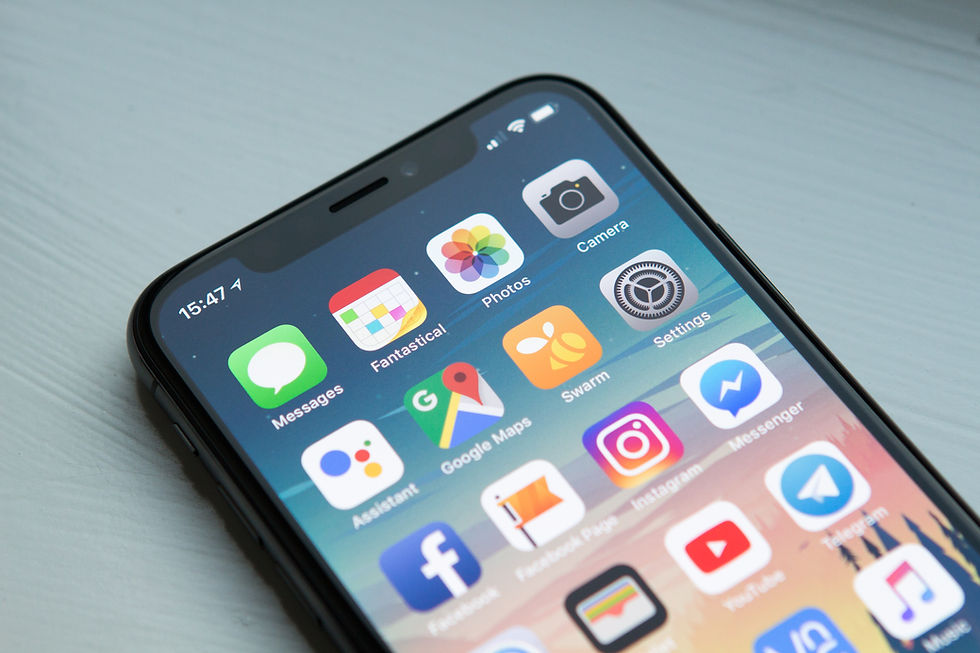
The basic app structure in Swift is very similar to the one you may know from Objective-C. When you create a new project using the "Single View Application" template, you will see two main files: AppDelegate.swift and ViewController.swift. Those of you who are familiar with iOS development, can see the resemblance to the equivalent files on Objective-C projects.
You can also find the methods that are called when the app is sent to/from the background, resigns/becomes active when a phone call is received, for example, and when the application is eventually terminated, removed from the background by the user or by the operating system.
In ViewController.swift, you can find the methods:
1. override func viewDidLoad()
2. override func didReceiveMemoryWarning()
The viewDidLoad() method is called (as its name suggests) when the view finishes loading. This is where you'll put necessary logic for the view's proper display.
The didRecieveMemoryWarning() method is a chance for you as the developer to clean things up if the operating system decides to warn you that your app is taking up too much space and may be terminated soon unless you take some action.
If you want to learn more about Mobile App development, CLICK HERE and enjoy!
4. Using Swift with Objective-c

If you have an existing Objective-C iOS project and don't want to completely migrate it to Swift, but still want to use some of Swift's advantages, you can mix and match. You can have both Swift and Objective-C files coexist in the same project, no matter what was the project's original language. All you need to do is create a new .swift file and add it to your existing Objective-C project.
If you're using both Swift and Objective-C files in the same project, you may want to access your Objective-C classes from your Swift code. In order to do so, you'll need to rely on an Objective-C bridging header.
When you add a file from a different programming language, either an Objective-C file to a Swift project or a Swift file to an Objective-C project, Xcode will offer to create such a bridging header file for you. In that file, you will need to import every Objective-C header you want to expose to Swift. All of the classes included in this file will be available in all Swift files without the need to import/include them in these files. Using these classes is done with the standard Swift syntax.
5. Safety
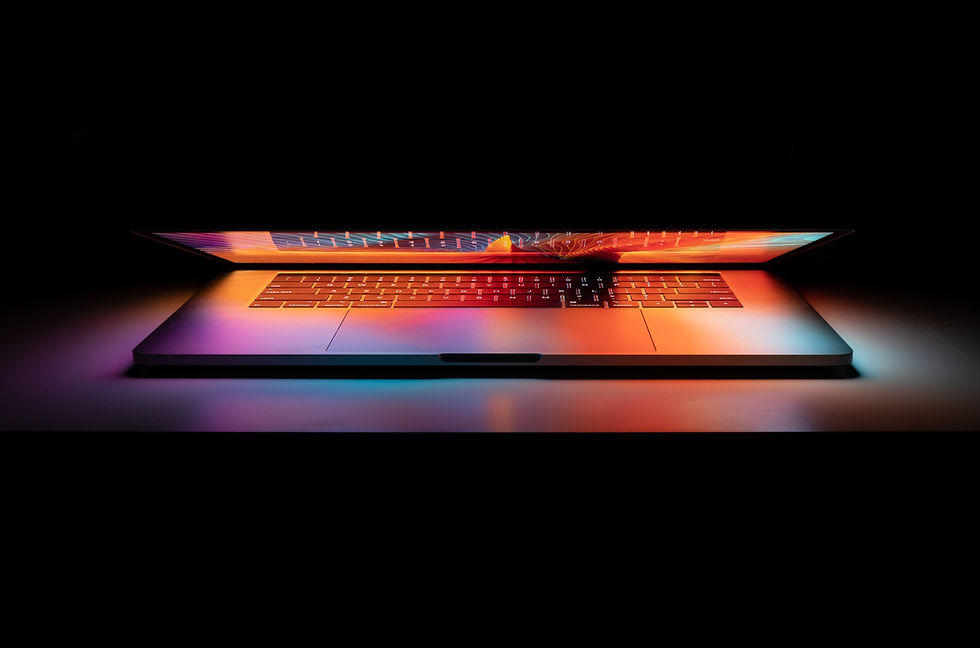
Swift eliminates entire classes of unsafe code. Variables are always initialized before use, arrays and integers are checked for overflow, memory is automatically managed and enforcement of exclusive access to memory guards against many programming mistakes. Syntax is tuned to make it easy to define your intent, for example, simple three-character keywords define a variable or constant. Swift heavily leverages value types, especially for commonly used types like Arrays and Dictionaries. This means that when you make a copy of something with that type, you know it won’t be modified elsewhere.
Another safety feature is that by default Swift objects can never be nil. In fact, the Swift compiler will stop you from trying to make or use a nil object with a compile-time error. This makes writing code much cleaner and safer and prevents a huge category of run time crashes in your apps. However, there are cases where nil is valid and appropriate. For these situations, Swift has an innovative feature known as "optionals". An optional may contain nil, but Swift syntax forces you to safely deal with it, using the '?' syntax to indicate to the compiler you understand the behavior and will handle it safely.
Comments